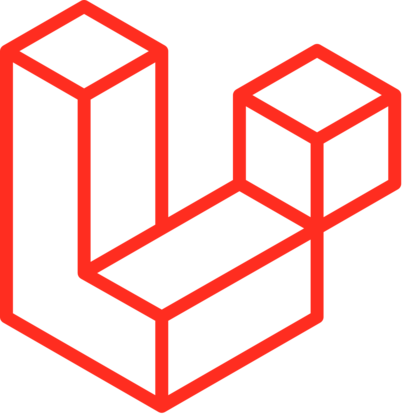
Cours de formation en ligne sur Google Meet + Cours au format PDF
25.00$
Compare
Installation environment
Installation Server, a complete turnkey solution for a high-performance server
Early Access
Learn about technologies in development
Guide Comprehensive training program
Train at your own pace with a range of guide setup available at any time.
Cloud-based workshops
Access the same workshops as in the environment room, 24/7, wherever you are.
-
SECTION – Prerequisites and Setup
-
An Animated Introduction to MVC
Before we get started, come along for a quick two minute overview of the MVC architecture. MVC stands for « Model, View, Controller » and is the bedrock for building Laravel applications.
-
Initial Environment Setup and Composer
I hope you’re excited. It’s time to dig in. Now, as for prerequisites, you’ll need access to a good editor, a terminal, and of course PHP and MySQL. We’ll also need to get a tool called Composer installed on your machine.
-
The Laravel Installer Tool
Extra Credit: Install Laravel Valet to make any new Laravel project accessible via http://app-name.test.
-
Why Do We Use Tools
Extra Credit: Consider watching the optional HTML and CSS Workflow prerequisite series that was mentioned in this video.
-
-
SECTION – The Basics
-
How a Route Loads a View
Let’s begin with the basics. If you load the home page for any new Laravel app in the browser, you’ll see a basic « welcome » splash page. In this lesson, we’ll figure out how a route « listens » for a URI and then loads a view (or HTML) in response.
-
Include CSS and JavaScript
Now that we understand how a particular URI ultimately loads a piece of HTML, let’s now figure out how to include some generic CSS and JavaScript assets.
-
Make a Route and Link to it
The simplest form of our blog will surely consist of a list of blog post excerpts, which then individually link to a different page that contains the full post. Let’s begin working toward that goal.
-
Store Blog Posts as HTML Files
Before we reach for a database, let’s discuss how to store each blog post within its own HTML file. Then, in our routes file, we can use a route wildcard to determine which post needs to be fetched and passed to the view.
-
Route Wildcard Constraints
Sometimes, you’ll wish to limit a route wildcard to only a certain sequence or pattern of characters. Luckily, Laravel makes this a cinch. In this episode, we’ll add a constraint to our route to ensure that the blog post slug consists exclusively of any combination of letters, numbers, dashes, and underscores.
-
Use Caching for Expensive Operations
Reaching for
file_get_contents()
each time a blog post is viewed isn’t ideal. Think about it: if ten thousand people view a blog post at the same time, that means you’re callingfile_get_contents()
ten thousand times. That surely seems wasteful, particularly when blog posts rarely change. What if we instead cached the HTML for each post to improve performance? Learn how in this episode. -
Use the Filesystem Class to Read a Directory
Let’s now figure out to fetch and read all posts within the
resources/posts
directory. Once we have a suitable array, we can then loop over them and display each on the main blog overview page. -
Find a Composer Package for Post Metadata
At the conclusion of the previous episode, we considered adding metadata to the top of each post file. As it turns out, this metadata format has a name: Yaml Front Matter. Let’s see if we can find a Composer package to help us parse it. This will give us a nice opportunity to learn how easy and useful Composer is.
-
Collection Sorting and Caching Refresher
Each post now includes the publish date as part of its metadata, however, the feed is not currently sorted according to that date. Luckily, because we’re using Laravel collections, tasks like this our a cinch. In this episode, we’ll fix the sorting and then discuss « forever » caching.
-
-
SECTION – Blade
-
Blade: The Absolute Basics
Blade is Laravel’s templating engine for your views. You can think of it as a layer on top of PHP to make the syntax required for constructing these views as clean and terse as possible. Ultimately, these Blade templates will be compiled to vanilla PHP behind the scenes.
-
Blade Layouts Two Ways
The next problem we need to solve relates to the fact that each of our views contains the full HTML structure – including any potential scripts and stylesheets. This means, should we need to add a new stylesheet, we must update every single view. This clearly won’t do. Instead, we can reach for layout files to reduce duplication. In this episode, I’ll demonstrate two different ways to create layouts.
-
A Few Tweaks and Consideration
Before we move on to the next chapter, on databases, let’s make a couple tweaks to wrap up these last two sections. First, we’ll remove the route constraint that is no longer required. Then, we’ll consider the benefits of adding a second
Post::findOrFail()
method that automatically aborts if no post matching the given slug is found.
-
-
SECTION – Working With Databases
-
Environment Files and Database Connections
Every application will require a certain amount of environment-specific configuration. Examples for this might be the name of the database you’re connecting to, or which mail host and port your app uses, or even special keys and secret tokens that third party APIs provide you. You can store configuration like this within your
.env
file, which is located in your project root. In this episode, we’ll discuss the essentials of environment files, and then move on to connecting to a MySQL database (using TablePlus). -
Migrations: The Absolute Basics
Now that we’ve properly connected to MySQL, let’s switch our attention over to those mysterious migration classes. Think of a migration as a blueprint for a database table.
-
Eloquent and the Active Record Pattern
Let’s now move on to Eloquent, which is Laravel’s Active Record implementation. Eloquent allows us to map a database table record to a corresponding Eloquent object. In this episode, you’ll learn the initial API – which should seem quite familiar if you followed along with the previous chapter.
-
Make a Post Model and Migration
Now that you’re a bit more familiar with migration classes and Eloquent models, let’s apply this learning to our blog project. We’ll remove the old file-based implementation from the previous chapter, and replace it with a brand new
Post
Eloquent model. We’ll also prepare a migration to build up theposts
table. -
Eloquent Updates and HTML Escaping
In this lesson, we’ll briefly discuss how to go about updating database records using Eloquent. Then, we’ll review an example of why escaping user-provided input is essential for the security of your application.
-
3 Ways to Mitigate Mass Assignment Vulnerabilities
In this lesson, we’ll discuss everything you need to know about mass assignment vulnerabilities. As you’ll see, Laravel provides a couple ways to specify which attributes may or may not be mass assigned. However, there’s a third option at the conclusion of this video that is equally valid.
-
Route Model Binding
Laravel’s route model binding feature allows us to bind a route wildcard to an Eloquent model instance.
-
Your First Eloquent Relationship
Our next job is to figure out how to assign a category to each post. To allow for this, we’ll need to create a new Eloquent model and migration to represent a
Category
. -
Show All Posts Associated With a Category
Now that we have the concept of a
Category
in our application, let’s make a new route that fetches and loads all posts that are associated with the given category. -
Clockwork, and the N+1 Problem
We introduced a subtle performance issue in the last episode that’s known as the N+1 problem. Because Laravel lazy-loads relationships, this means you can potentially fall into a trap where an additional SQL query is executed for every item within a loop. Fifty items…fifty SQL queries. In this episode, I’ll show you how to debug these queries – both manually, and with the excellent Clockwork extension – and then we’ll solve the problem by eager loading any relationships we’ll be referencing.
-
Database Seeding Saves Time
In this lesson, we’ll associate a blog post with a particular author, or user. In the process of adding this, however, we’ll yet again run into the issue of needing to manually repopulate our database. This might be a good time to take a few moments to review database seeding. As you’ll see, a bit of work up front will save you so much time in the long run.
-
Turbo Boost With Factories
Now that you understand the basics of database seeders, let’s integrate model factories in order to seamlessly generate any number of database records.
-
View All Posts By An Author
Now that we can associate a blog post with an author, the next obvious step is to create a new route that renders all blog posts written by a particular author.
-
Eager Load Relationships on an Existing Model
In this episode, you’ll learn how to specify which relationships should be eager loaded by default on a model. We’ll also touch on the pros and cons of such an approach.
-
-
SECTION – Integrate the Design
-
Convert the HTML and CSS to Blade
Extra Credit: Consider watching the optional HTML and CSS Workflow prerequisite series, where we write the HTML and CSS that is referenced in this chapter.
-
Blade Components and CSS Grids
We’re making great progress. Let’s continue the conversion in this episode, as we take a break from Laravel to play around with CSS grids.
-
Convert the Blog Post Page
With the home page in reasonably good shape, let’s now move on to the « view blog post » page and get that up and running.
-
A Small JavaScript Dropdown Detour
We next need to make that « Categories » dropdown on the home page function as expected. I hate to break it to you, but we’ll need to reach for a bit of JavaScript to make this work. Don’t worry: I’ll make this as painless as possible by pulling in the excellent Alpine.js library. Let’s get through this, and we’ll jump back into some Laravel-specific topics!
-
How to Extract a Dropdown Blade Component
We’ve now successfully built the basic functionality for a dropdown menu, but it’s not yet reusable. To remedy this, let’s extract an
x-dropdown
Blade component. This will come with the side effect of isolating all Alpine-specific code to that single component file. -
Quick Tweaks and Clean-Up
We’re about to move on to the search functionality, but before we do that, let’s take five minutes to do some quick clean up.
-
-
SECTION- Search
-
Search (The Messy Way)
In this new section, we’ll implement the search functionality for our blog. I’m going to demonstrate this in two steps. First, in this video, we’ll simply get it to work. The code won’t be reusable or pretty, but it’ll work! Then, in the following episode, we can refactor a bit.
-
Search (The Cleaner Way)
Now that our search form is working, we can take a few moments to refactor the code into something more pleasing to the eye (and reusable). In this episode, not only will we finally have a look at controller classes, but we’ll also learn about Eloquent query scopes.
-
-
SECTION – Filtering
-
Advanced Eloquent Query Constraints
Let’s keep playing with our
Post
model’sfilter()
query scope. Maybe we can additionally filter posts according to their category. If we take this approach, we’ll then have a powerful way of combining filters. For example, « give me all posts, written by such-and-such author, that are within the given category, and include the following search text.« -
Extract a Category Dropdown Blade Component
Have you noticed that each route needs to pass a collection of categories to the
posts
view? If you take a look, that variable is only ever referenced as part of the main category dropdown. So with that in mind, what if we created a dedicatedx-category-dropdown
component that could be responsible for fetching any data that the dropdown requires? Let’s figure out how to allow for that in this episode. -
Author Filtering
Let’s next add support for filtering posts according to their respective author. With this final piece of the puzzle, we’ll now be able to easily sort all posts by category, or author, or search text, or all of the above.
-
Merge Category and Search Queries
We next need to update both the category dropdown and search input to include all existing and relevant query string parameters. Right now, if we’re browsing a certain category, as soon as we perform a search, that current category will revert back to « All. » Let’s fix that.
-
Fix a Confusing Eloquent Query Bug
It looks like we have a slight error within our
filter()
query scope. In this lesson, we’ll review the underlying SQL query that’s producing the incorrect results, and then fix the bug in our Eloquent query.
-
-
SECTION – Pagination
-
Laughably Simple Pagination
We’re currently fetching all posts from the database and rendering them as a grid on the home page. But what happens down the line when you have, say, five hundred blog posts? That’s a bit too costly to render. The solution is to leverage pagination – and luckily, Laravel does all the work for you. Come see for yourself.
-
-
SECTION – Forms and Authentication
-
Build a Register User Page
We’ve put it off long enough: it’s time to move on to form handling and user authentication. To begin, let’s create a route that displays a registration form to sign up for our site.
-
Automatic Password Hashing With Mutators
We ended the previous episode with a cliffhanger: passwords were being saved to the database in plain text. We can never allow this. Luckily, the solution is quite easy. We’ll leverage Eloquent mutators to ensure that passwords are always hashed before being persisted.
-
Failed Validation and Old Input Data
We next need to provide the user with feedback whenever the validation checker fails. In these cases, we can reach for the
@error
Blade directive to easily render an attribute’s corresponding validation message (if any). We’ll also discuss how to fetchold()
input data. -
Show a Success Flash Message
We aren’t yet providing the user with any feedback after they register on our site. Let’s fix that by displaying a one-time flash message.
-
Login and Logout
The final piece of the puzzle is the actually log the user in. We can once again reach for either the
auth()
helper function (or facade) to perform thelogin()
andlogout()
actions. -
Build the Log In Page
We wrapped up the previous episode by creating a Log In link, but we stopped just short of building the view. Let’s tackle that now.
-
Laravel Breeze Quick Peek
Because we’ve already spent some time working on a custom authentication system for our blog, we’ll go ahead and stick with. it. HHowever, we should still set aside a few moments to quickly review Laravel’s excellent first-party authentication packages. Specifically, in this episode, we’ll take a peek at Laravel Breeze.
-
-
SECTION – Comments
-
Write the Markup for a Post Comment
Let’s now move on to post comments. We’ll begin with the base markup for a comment.
-
Table Consistency and Foreign Key Constraints
We can next move on to building up the migration and corresponding table for our comments. This will give us a chance to more deeply discuss foreign key constraints.
-
Make the Comments Section Dynamic
Now that we have a comments table ready to go, let’s now switch over and build up the necessary attributes for our
CommentFactory
. Once complete, we’ll then switch back to our post page and make the comments section loop over what we have stored in the database. -
Design the Comment Form
Now that each post can display a list of comments, let’s next create a form to allow any authenticated user to participate in the conversation.
-
Activate the Comment Form
Now that the comment form is fully designed, we can add the necessary logic to « activate it. »
-
Some Light Chapter Clean Up
Before we move on to the next section, let’s take a few moments to clean up after ourselves. We’ll extract a couple Blade components, create a PHP include, and then manually reformat bits of our code.
-
-
SECTION – Newsletters and APIs
-
Mailchimp API Tinkering
Let’s begin by familiarizing ourselves with the Mailchimp API. We’ll learn how to install the official PHP SDK, and then review the basics of how to make some initial API calls.
-
Make the Newsletter Form Work
Okay, now that we understand the basics of how to add an email address to a Mailchimp list, let’s update the newsletter form.
-
Extract a Newsletter Service
I think we’re comfortable enough with this small piece of code to extract it into its own
Newsletter
service class. -
Toy Chests and Contracts
This next episode is supplementary. It’s slightly more advanced, and reviews service containers, providers, and contracts. Though I do my best to break it all down, please feel free to ask any questions you might have in the comments below. Otherwise, if you feel left behind by this episode, the truth is you can sneak by for a long time without fully understanding these concepts.
-
-
SECTION – Admin Section
-
Limit Access to Only Admins
Let’s finally work on the administration section for publishing new posts. Before we begin constructing the form, let’s first figure out how to add the necessary authorization layer to ensure that only administrators may access this section of the site.
-
Create the Publish Post Form
Okay, now that we’ve taken a first stab at adding some route authorization, let’s now finish building the « Publish Post » form.
-
Validate and Store Post Thumbnails
In this video, you’ll learn how to upload images to your local disk using a standard file input and Laravel’s
UploadedFile
class. It’s so easy! -
Extract Form-Specific Blade Components
In this video, you’ll learn how to clean up the HTML for a form by extracting a series of reusable « pieces » that can be used to construct each section. We’ll of course use Blade components to allow for this.
-
Extend the Admin Layout
In this episode, we’ll add an account dropdown menu to the navigation area, and then extend the layout for our settings/adadministration section to allow for a sidebar.
-
Create a Form to Edit and Delete Posts
There’s one glaring feature missing that we need to implement: any post may be edited or deleted. We’ll work on allowing for that in this episode.
-
Group and Store Validation Logic
You’ll notice that our controller validation logic has now been mostly duplicated. In this lesson, we’ll discuss the pros and cons of keeping that duplication, before learning how to normalize and extract it into a reusable method.
-
All About Authorization
Did you notice that anyone who is signed in will currently see admin-specific links within the dropdown navigation bar? How might we fix that? It sounds like we’ll need to rework our authorization just a bit.
-
-
SECTION – Conclusion
-
Goodbye and Next Steps
All things must come to an end, including this very long series. So congratulations if you made it this far. In this final goodbye video, we quickly review what you’ve learned, as well as some recommended
-